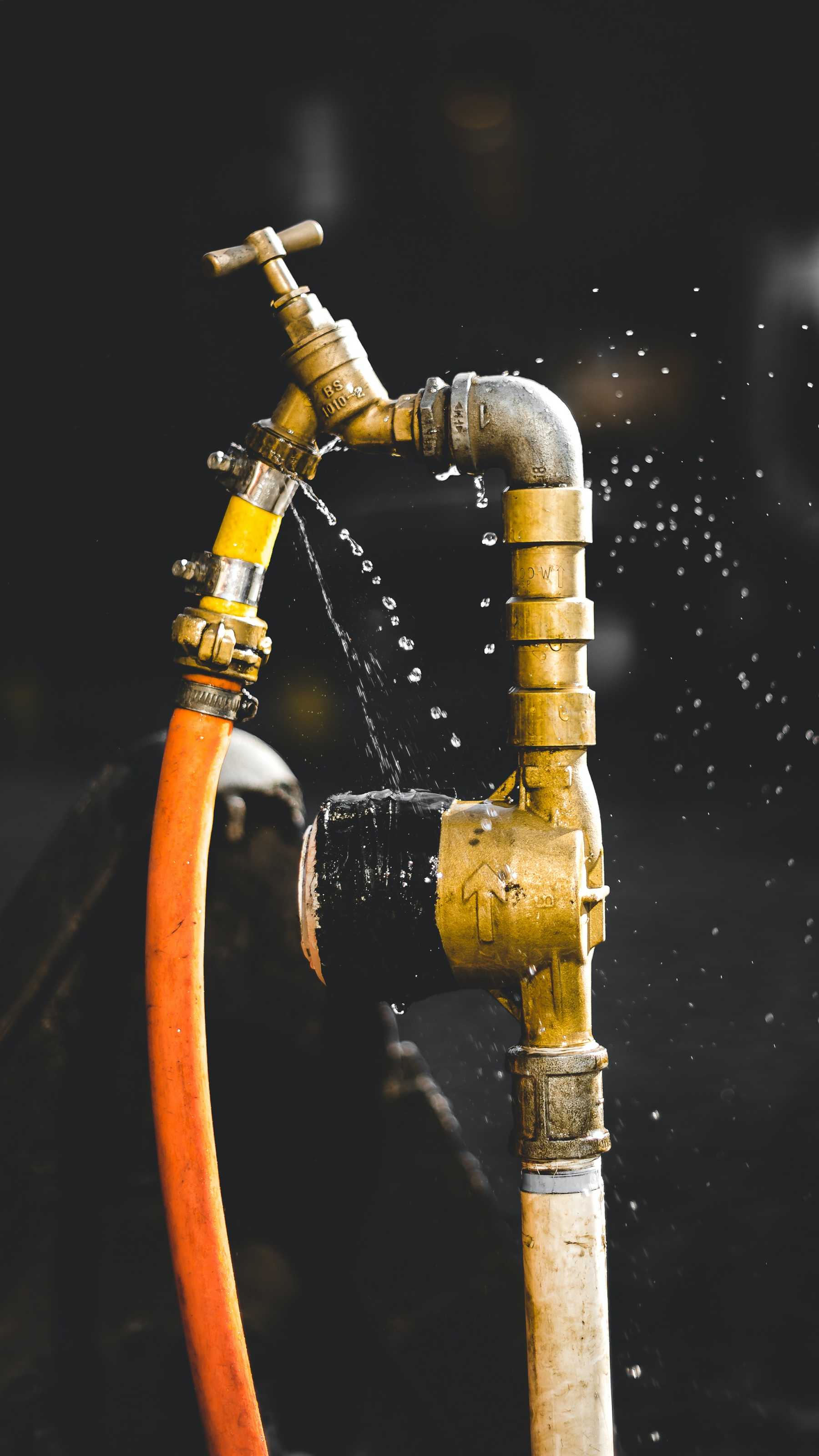
Django REST Framework 101 - Reading Time: 5 Mins
📅 April 12, 2020
•⏱️6 min read
Introduction
In this article, I hope to help you to learn to get started with building API using the Django REST Framework. I found that there is a lot of things to consider when creating an API that even includes an API development life cycle for creating an API. From the API design & mocking to documenting the API for widespread adoption for other developers/organisations to integrate the API into their products or services.
If you are interested in creating APIs for a living and the ecosystem. i would suggest that you go to API Evangelist. As he spends tons of time and effort to cover the API ecosystem.
Which from what I know is a big industry if you don't believe me take a look at Postman & Kong latest fundraising. This becomes bigger as large organisations, financial institutions focus on building microservices, using GraphQL and having policies like open data as part of their strategy. For others to adopt to create products or services on top of existing data or APIs.
Project Layout
I won't be covering the setup process and creation of the Django. If you like to refer to it. Please go to the Django REST Framework Quickstart on how to do it. I will be referring directly from it with additional information and explanation to make it easy for you to understand:
Project Structure
.
./manage.py
./tutorial
./tutorial/__init__.py
./tutorial/quickstart
./tutorial/quickstart/__init__.py
./tutorial/quickstart/admin.py
./tutorial/quickstart/apps.py
./tutorial/quickstart/migrations
./tutorial/quickstart/migrations/__init__.py
./tutorial/quickstart/models.py
./tutorial/quickstart/tests.py
./tutorial/quickstart/views.py
./tutorial/settings.py
./tutorial/urls.py
./tutorial/wsgi.py
Serializers
Serializers is data representation for your API which pulls from your models
that are created by you or prebuilt with Django. Think of it as a template on how your data is shown in an excel spreadsheet. Where the first row of each column represents a specific field for a row:
serializers.py
# Django's prebuilt models for user & group
from django.contrib.auth.models import User, Group
from rest_framework import serializers
"""
Packages the models into a format that could be represented in an API
The HyperlinkedModelsSerializer provides an actual link to the record of the database when you would like to access that specific record
"""
class UserSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = User
# Types of fields for the User model
fields = ['url', 'username', 'email', 'groups']
"""
Packages the models into a format that could be represented in an API
"""
class GroupSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = Group
# Types of fields for Groups model
fields = ['url', 'name']
Views
In Django, views are used as the presentation layer which you combine it with Django's built-in template engine to create the webpage when a view is called.
Whereas in Django REST Framework (DRF), you can code the response yourself. But DRF provides many prebuilt views that are similar to Django forms to cover basic needs required for a developer.
One of them is called ModelViewSet
which as the name suggested presents data directly from your models
that created by you or prebuilt by Django. The other is called permission
, which only allows the correct user with the right permission
to access the API.
For further details about the various viewset
you can head to Viewsets in the DRF documentation which lists down the various prebuilt viewset for you to use:
My suggestion for you when you are using DRF's prebuilt viewsets is that you stick to the prebuilt viewsets. A word of caution, it can be quite painful to customise it. Unless you know know what you are doing without the compromising the security of the API by reading the documentation carefully to not invite security loopholes to it:
views.py
from django.contrib.auth.models import User, Group
# prebuilt view to present the model based upon the serializer used.
from rest_framework.viewsets import ModelViewSet
from rest_framework.permissions import IsAuthenticated
from tutorial.quickstart.serializers import UserSerializer, GroupSerializer
class UserViewSet(ModelViewSet):
"""
API endpoint that allows users to be viewed or edited.
"""
# Django ORM which selects all database records based upon
# their join date in descending order
queryset = User.objects.all().order_by('-date_joined')
serializer_class = UserSerializer
# Checks if the user has the right permission to acccess the data
permission_classes = [IsAuthenticated]
class GroupViewSet(ModelViewSet):
"""
API endpoint that allows groups to be viewed or edited.
"""
queryset = Group.objects.all()
serializer_class = GroupSerializer
# Checks if the user has the right permission to acccess the data
permission_classes = [IsAuthenticated]
URLs
Since you had spent the time & effort in creating both the serializer
& view
for your Django REST API. Now is the time for you to create the URL
where the router will redirect you to the API whenever you initiate an API request for it:
urls.py
from django.urls import include, path
from rest_framework import routers
from tutorial.quickstart import views
"""
Creates a router that redirects to various views
"""
router = routers.DefaultRouter()
# To access the API it uses "users" as part of URL for the API
# which the url is http://127.0.0.1:8000/users/
router.register(r'users', views.UserViewSet)
# To access the API it uses "group" as part of URL for the API
# which the url is http://127.0.0.1:8000/groups/
router.register(r'groups', views.GroupViewSet)
"""
Wire up our API using automatic URL routing.
Additionally, we include login URLs for the browsable API.
"""
urlpatterns = [
# This is put as the "root url" for your newly created APIs which your
# router routes to the various views that was registered by you.
path('', include(router.urls)),
# prebuilt DRF login/logout views to authenticate the user
path('api-auth/', include('rest_framework.urls', namespace='rest_framework'))
]
Conclusion
I hope it provides an overview for you to start building your first API using the Django REST Framework. Along with my exploration and understanding of it to help you to start creating. I know that it might seem to be quite overwhelming and is not as straight forward or easy when you are comparing it with Flask or ExpressJS.
What I could assure it is that the DRF is quite a well thought which reduces the amount of work tremendously if you understand it well.
The key reason that I would stick to Django REST framework is when I am building something from the ground up. With specific requirements for security, scalability & ease of maintaining. Due to Django's opinionated approach in building APIs or websites with it, unlike Flask or ExpressJS.